CSS Hack: Disable Chrome’s Pull-to-Refresh on Android
I was working on a small project for a friend lately. One of the needs of the project was to get rid of Google Chrome’s Pull-to-refresh functionality on certain web pages. This post wraps up how I did it with just CSS.
About Pull-to-refresh on Chrome for Android
When being at the very top of a web page on Chrome on your Android device, you may reload it by tap-holding, and swiping down. Of course, you may turn this feature off for all the tabs by visiting chrome://flags/#disable-pull-to-refresh-effect on your chrome browser, and then set it to Disable.
Update: Some of our reader friends reminded me that the flag I mentioned above is removed from Chrome 75+. I have the pull-to-refresh flag working fine in my Chrome with build 75.0.3770.100, but it has stopped showing up in 75.0.3770.101.
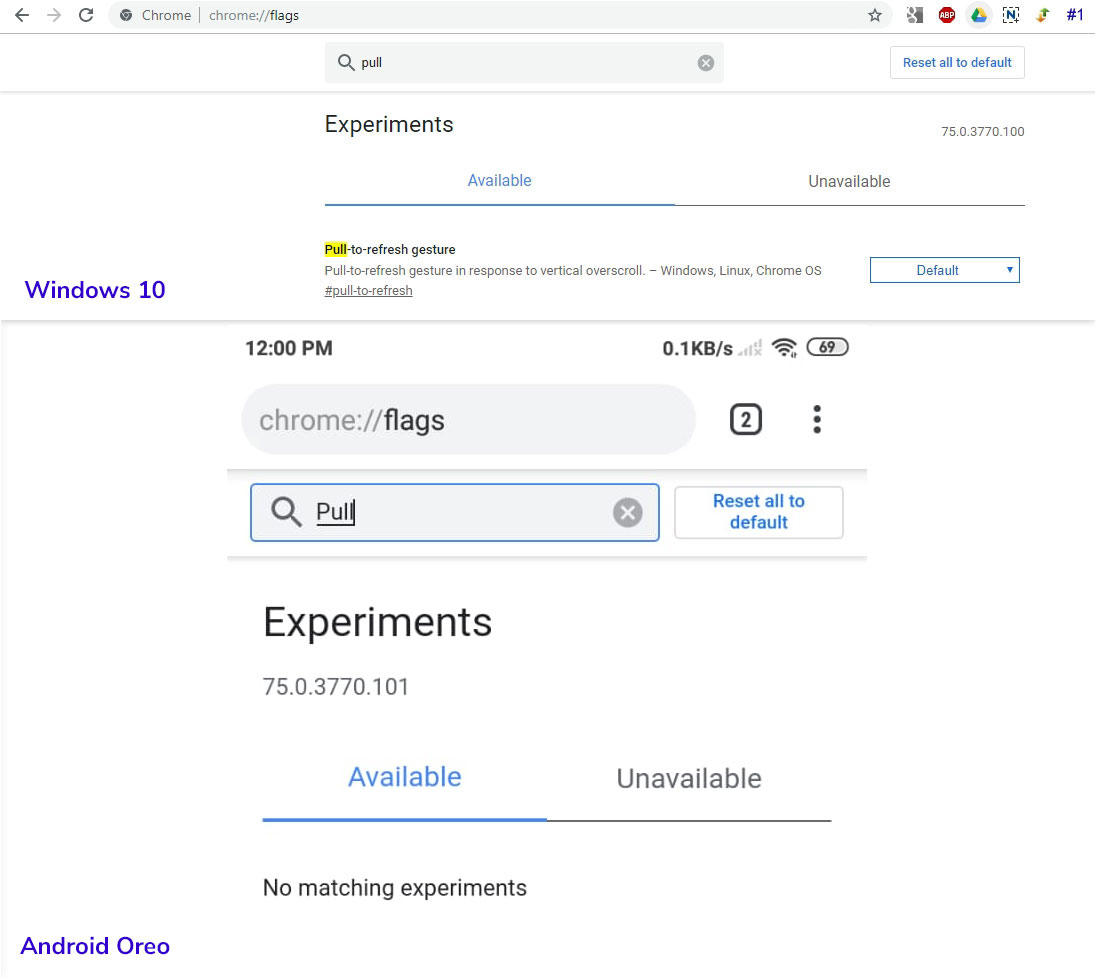
Little unfortunate, I don’t know yet what else could be a solution to this problem.
But, asking your users to do that in order to make your page functional to them sounds ridiculous. And this is where the need of some CSS/JS tweaks jumps in.
Where do you need to override it?
Let’s say you have an iframe embedded on the topmost section of the webpage that has some dynamic content to load on scrolling up and down (mine was the same case). Here, the pull-to-refresh functionality of Chrome may intervene and spoil the user experience. That’s just an example, your reason could be different than mine.
You might already know about CSS overscroll-behavior
property, that allows you to do that in just one line of code on Chrome 63+:
.wrapper { overscroll-behavior: none }
If you are interested to know more about overscroll-behavior
property, you should read this Google guide for a detailed overview about the same.
Looking to make it work everywhere and not just on Chrome 63+? Read on for the trick which may also be used as a polyfill for this workaround of overscroll-behavior
.
Temporarily disabling Pull-to-Refresh: The trickery
By temporarily, I mean disabling it just for your website. I don’t know yet how to achieve it with JavaScript, but I did figure out what CSS can do for me in this case.
The trick lies in taking the body and it’s inside container division out of the document flow while maintaining the element stacking. That’s it.
CSS
.body,
.wrapper {
/* Break the flow */
position: absolute;
top: 0px;
/* Give them all the available space */
width: 100%;
height: 100%;
/* Remove the margins if any */
margin: 0;
/* Allow them to scroll down the document */
overflow-y: hidden;
}
.body {
/* Sending body at the bottom of the stack */
z-index: 1;
}
.wrapper {
/* Making the wrapper stack above the body */
z-index: 2;
}
HTML
<!doctype html>
<html>
<head>
<!-- Put the above styles here -->
</head>
<body class="body">
<div class="wrapper">
<!-- Put the page contents here -->
</div>
</body>
</html>
Note that content you wish to put in the document goes inside the .wrapper
. Check out the demo on your Chrome for Android using below link, and try pull-to-refresh, and let me know if the above CSS was successful or not.
Note that I’ve embedded this website’s homepage in the iframe
for the demonstration.
Should you have any queries and suggestions regarding the above stuff, feel free to use the comment section below to let me know about your thoughts.
Load Comments...